A Skill Tree for Learning Computer Science
Mapped out with LeetCode Explore Cards
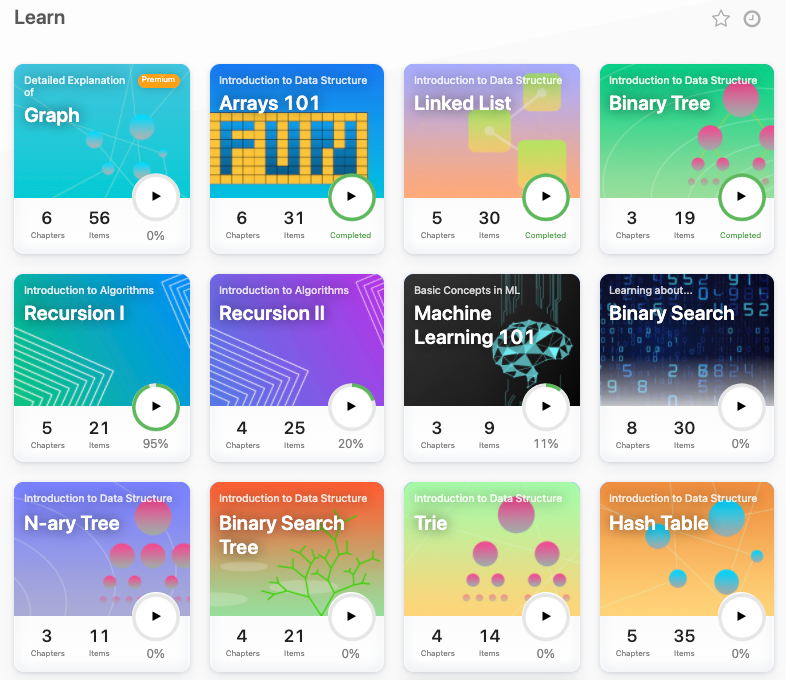
I assume that you’ve already picked a programming language, whether it is Python, Java, C++, or a different one. You don’t need to have an in-depth understanding of the nuances of your chosen programming language, you only need to have a basic understanding of syntax and programming concepts (loops and data types). I also assume that you are here to figure out the order in which to learn the different data structures and algorithms without having to take courses at a university.
(Disclaimer: some of these cards, questions, and solutions require a Premium subscription to access.)
The Start
What are the simplest data structures? Arrays and Strings
While we consider Arrays and Strings, we also have another explore card that will go through more problems concerning Arrays: Arrays 101
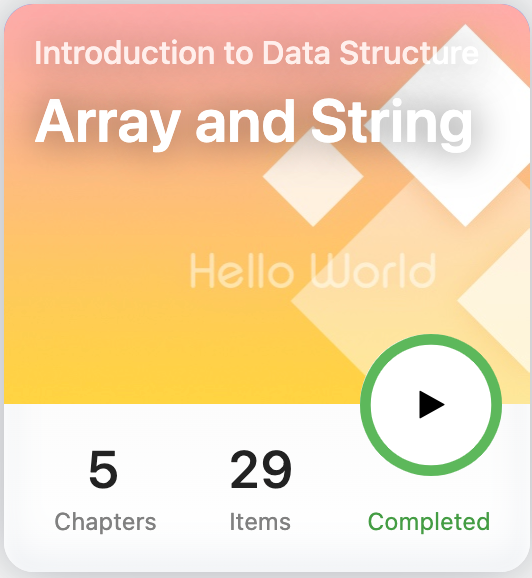
After we finish learning about Arrays and Strings, we can start learning about a different type of list: A Linked List. Linked Lists are similar to Arrays or Array Lists in that it is a linear data structure. However, they are built with nodes that have pointers that connect each node to the next within the list.
After we’re done thinking about Linked Lists, — both the singly-linked ones and the doubly-linked ones — we can now expand the idea of nodes and pointers to that of a Tree, specifically a Binary Tree. Binary Trees grow from a root node and follow the rule that each node can only point to a maximum of 2 other nodes. From here, we can consider algorithms that relate to Binary Trees, like Binary Search with the Binary Search Tree.
The simplest Binary Search problems only require knowledge of Arrays and Strings.
Intermediate Stage
Here, we can venture into slightly more involved data structures and algorithms. The explore cards here may take more time to fully complete and understand. I’ve also found that the cards at this step are best done in combination as knowledge of data structures can help solve algorithmic Explore cards, and vice versa.
The notable data structure cards at this stage include Hash Table(s), Queue(s) and Stack(s), and the Heap. Hash Tables, Queues, and Stacks are the most simple data structures after understanding Arrays. In fact, in most languages, Queues and Stacks can be implemented using Arrays. Although, dedicated object classes can be built for these data structures if desired.
The algorithms-related card that I would classify as intermediate is Recursion I. However, I will note that some of the problems in the previously mentioned Explore Cards can be implemented with recursion instead of iteration. Other algorithms in this stage include graph search algorithms like DFS (Depth-First Search) and BFS (Breadth-First Search). These are covered in the Queue and Stack card.
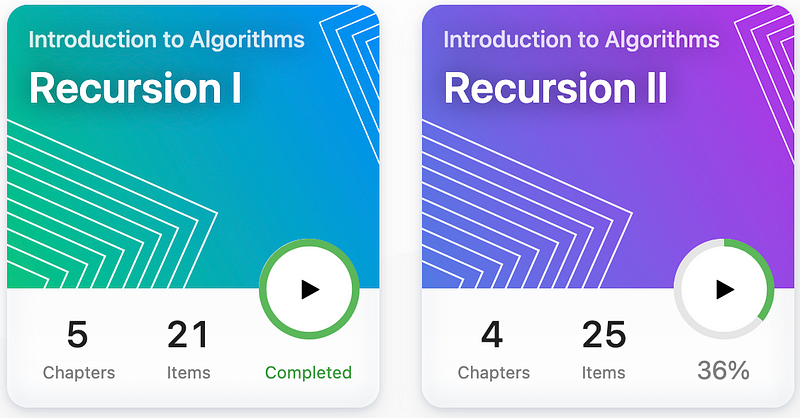
Advanced Stage
The rest of the cards that I haven’t mentioned would belong in the Advanced stage. I like to split these into 2 categories, “the strongly recommended” and “the less common”.
“The strongly recommended” cards include the Graph card, in which the Union-Find data structure is covered, Dynamic Programming, and Recursion II. I find that Recursion II and Dynamic Programming go hand-in-hand as DP is often implemented recursively, and solutions may involve backtracking (which is covered in Recursion II). Other algorithms covered in Recursion II include Divide and Conquer. Completing the Explore cards up to this point may be enough to complete a majority of technical coding challenges. This is also the point at which most undergraduate Computer Science courses finish.
The other “less common” cards that I’d like to mention are N-ary Tree and Trie, a specific type of N-ary Tree. These are more advanced continuations after Binary Tree, as they are other more complex types of tree structures.
I’d also like to give an honorary shoutout to the Machine Learning cards Machine Learning 101 and Decision Tree, as these may be useful to those who want to venture into Machine Learning Engineering or Data Science positions.
Extra Notes
Completing this LeetCode path does not guarantee you a software engineering job, which is what I assume most LeetCoders have in mind as a goal. Software Engineering also requires system design, a topic that LeetCode does not cover. That said, I hope that my LeetCode path helps others who are interested in a programming or Software Engineering career start their journey to learn (or review), their algorithms and data structures.
Good luck and have fun!